C ダウンキャスト dynamic_cast 345443
· C dynamic_cast Performance ( Updated Rerun the test with latest clang from subversion ) A performance comparison of the speed of dynamic_cast operations in CDynamic_cast doesn't have the ability to remove a const qualifier You can cast away const separately using a const_cast, but it's generally a bad idea in most situationsFor that matter, if you catch yourself using dynamic_cast, it's usually a sign that there is a better way to do what you are trying to do · dynamic_cast演算子 動的なバインディングこと、実行時にcastができるCの機能だよ。 なにかとweb上のdynamic_cast演算子の項目には「ダウンキャスト」という単語がちらほらでてくる。 基底classから派生classへのcastのことを「ダウンキャスト」というそうで。
C のダウンキャストについて考える 見切り発車
C ダウンキャスト dynamic_cast
C ダウンキャスト dynamic_cast-Dynamic_cast is part of the RunTime Type Information (RTTI) feature that provides a way to access the type of an object at run time instead of compile time Mind that dynamic_cast can not be used to convert between the primitive types like int or floatDynamic_cast < T > (v) With the right angle bracket feature, you may specify a template_id as T in the dynamic_cast operator with the >> token in place of two consecutive > tokens For details, see Class templates (C only)
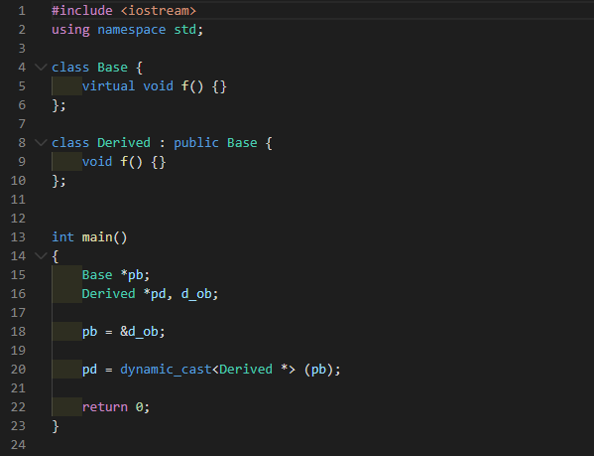


Dynamic Castについて うどたくのフック
When should static_cast, dynamic_cast, const_cast and reinterpret_cast be used in C?Dynamic_cast to a pointer to the underlying type of a boxed enum will fail at runtime, returning 0 instead of the converted pointer dynamic_cast will no longer throw an exception when typeid is an interior pointer to a value type, with the cast failing at runtime The cast will now return the 0 pointer value instead of throwing1712 · 6) When dynamic_cast is used in a constructor or a destructor (directly or indirectly), and expression refers to the object that's currently under construction/destruction, the object is considered to be the most derived object
Cのキャストで使われるdynamic_cast(動的なキャスト)は、安全にダウンキャストを行います。 正しくないポインタを指定してダウンキャストしたとき、キャストの結果は NULL となります。概要 shared_ptr で管理するインスタンスに対して dynamic_cast を行う。 戻り値 r が空であった場合、この関数は空の shared_ptr を返却する。 (1)What is the difference between static_cast and C style casting?
Why use static_cast(x) instead of (int)x in C?If dynamic_cast is used to convert to a reference type and the conversion is not possible, an exception of type bad_cast is thrown instead dynamic_cast can also perform the other implicit casts allowed on pointers casting null pointers between pointers types (even between unrelated classes), and casting any pointer of any type to a void* pointerUse dynamic_cast() as a function, which helps you to cast down through an inheritance hierarchy (main description) If you must do some nonpolymorphic work on some derived classes B and C , but received the base class A , then write like this


C のモヤモヤをデバッグで解消 キャスト編 Insight Technology



Google C スタイルガイドを読んで知らないことを調べたメモ 学習b5デスノート
The qobject_cast() function behaves similarly to the standard C dynamic_cast(), with the advantages that it doesn't require RTTI support and it works across dynamic library boundaries It attempts to cast its argument to the pointer type specified in anglebrackets, returning a nonzero pointer if the object is of the correct type (determined非常に大規模なC コードベース(Mozilla、OpenOfficeなど)では、RTTIを無効にする (したがって、 dynamic_castと例外を使用できない)という習慣があります。これは、 RTTIデータを実行可能ファイルに含めるだけのオーバーヘッドは許容できないためです。特に、動的な再配置が追加されるDynamic_cast on vector in C Before going to code we need to be clear with the syntax of dynamic_cast and working of it And dynamic_cast only works when we have at least one virtual function in the base class That is dynamic_cast works whenever there is a polymorphism Syntax dynamic_cast(expression) Code1)



C のキャスト 変換処理 を結婚に例えると Boost株式会社 毎日を ゲームに



実線c 入門講座 第33回目 C の型変換でバグを未然に防ごう Theolizer
0904 · Concepts library (C) Diagnostics library Utilities library Strings library Containers library Iterators library Ranges library (C) Algorithms library Numerics library Localizations library Input/output library Filesystem library (C17) Regular expressions library (C11) Atomic operations library (C11) Thread support libraryRegular cast vs static_cast vs dynamic_cast in C program;2112 · C provides a casting operator named dynamic_cast that can be used for just this purpose Although dynamic casts have a few different capabilities, by far the most common use for dynamic casting is for converting baseclass pointers into derivedclass pointers This process is called downcasting Using dynamic_cast works just like static_cast


C のモヤモヤをデバッグで解消 キャスト編 Insight Technology


ダウンキャストに潜む罠 Syghの新フラグメント置き場
Jeżeli chodzi o dynamic_cast, to oczywiście (jak wszystko w C) jest to bardzo elastyczne narzędzie Większość osób (moim przypuszczeniem) nie zna pełni możliwości dynamicznego rzutowania, ponieważ albo nigdy takiej funkcjonalności nie potrzebowali, albo nigdy po prostu nie było dane im poznanie jakiegoś konkretnego zachowaniaDynamic_cast 会在程序运行期间借助 RTTI 进行类型转换,这就要求基类必须包含虚函数;static_cast 在编译期间完成类型转换,能够更加及时地发现错误。 dynamic_cast 的语法格式为: dynamic_cast (expression) newType 和 expression 必须同时是指针类型或者引用类型。2705 · dynamic_cast or to make it polymorphic You need to have a polymorphic class to use dynamic_cast IN THIS FASHION The presence of the virtual function in the base class is what makes it "polymorphic" by DEFINITION



Abstract Factory



C のモヤモヤをデバッグで解消 キャスト編 Insight Technology
Char* c = reinterpret_cast(&j);Dynamic_cast 演算子が正常に行われると、arg によって表されるオブジェクトを指すポインターを戻します。 dynamic_cast が失敗すると、 0 が戻されます。 downcast は、ポリモアフィック・クラスにおいてのみ、 dynamic_cast 演算子を使用して、 実行することができます。演算子は、 dynamic_cast "クロスキャスト" を実行するためにも使用できます。 同じクラス階層を使用すると、完全なオブジェクトが B 型であれば、たとえば D サブオブジェクトから E サブオブジェクトへのように、ポインターをキャストできます。



アップキャストとダウンキャスト Syntax Error



C のキャスト 変換処理 を結婚に例えると Boost株式会社 毎日を ゲームに
111 static_cast、reinterpret_cast、const_cast 和 dynamic_cast 将类型名作为强制类型转换运算符的做法是C语言的老式做法,C 为保持兼容而予以保留。 C 引入了四种功能不同的强制类型转换运算Dynamic cast of shared_ptr Returns a copy of sp of the proper type with its stored pointer casted dynamically from U* to T* If sp is not empty, and such a cast would not return a null pointer, the returned object shares ownership over sp 's resources, increasing by one the use count Otherwise, the returned object is an empty shared_ptrDynamic_cast in C can be used to perform type safe down casting dynamic_cast is run time polymorphism The dynamic_cast operator, which safely converts from a pointer (or reference) to a base type to a pointer (or reference) to a derived type eg 1



Atlasjapansoftwaretutorial Main Twiki


セール バスタオル ワッフル バスタオル Lbc エルビーシー のファッション インテリア バスタオル
Shows the differences between C static_cast and dynamic_cast · C Tricks is a series of posts on core libraries for game engines and language experiments shared as the Kahncode Core Libraries As introduced in the first post of these series, I will share the first piece of KCL an implementation of RTTI and Dynamic CastThe code can be found on GitHub If you don't know what dynamic casting is, then I suggest you read some0519 · Regular cast vs static_cast vs dynamic_cast in C;



15 号 オンラインビルドシステム オンラインビルド方法およびオンラインビルドプログラム Astamuse



新作製品 世界最高品質人気 ホビー B0q51wyh 激安超特価 正規品送料無料 100 品質保証 100 品質保証 車 バイク 子供用漫画ヘルメット 女の子 男の子 年齢3 8 動物モデルの安全サイクリング スケートボード Bmxスポーツヘルメット 取り外し可能なライナー
This video lecture is produced by S Saurabh He is BTech from IIT and MS from USAdynamic_cast in cdynamic casting in c pptstatic and dynamic casting iDynamiccast Typecast Dynamic casts are only available in C and only make sense when applied to members of a class hierarchy ("polymorphic types") Dynamic casts can be used to safely cast a superclass pointer (or reference) into a pointer (or reference) to a subclass in a class hierarchy If the cast is invalid because the the real type ofTry this const Der* der = dynamic_cast(base);


C のモヤモヤをデバッグで解消 キャスト編 Insight Technology


39項 継承の階層構造を下る方向のキャスト ダウンキャスト は避けよう プログラミングメモ
The dynamic_cast operator is intended to be the most heavily used RTTI component It doesn't give us what type of object a pointer points to Instead, it answers the question of whether we can safely assign the address of an object to a pointer of a particular type Unlike other casts, a dynamic_cast involves a runtimeC also contains the type conversion operators const_cast, static_cast, dynamic_cast, and reinterpret_cast The formatting of these operators means that their precedence level is unimportant Most of the operators available in C and C are also available in other Cfamily languages such as C#, D,C では,次の 4 つの名前付きキャスト演算子が導入されました。 静的キャスト (static_cast) 動的キャスト (dynamic_cast) const キャスト (const_cast) 再解釈キャスト (reinterpret_cast) これらのキャストは,キャスト名(式) の形で用います。


お気楽c プログラミング超入門


ダウンキャスト Void Pないと
· dynamic_cast This cast is used for handling polymorphism You only need to use it when you're casting to a derived class This is exclusively to be used in inheritance when you cast from base class to derived class第42章 ダウンキャスト 前回に続いて、今回もC++のキャストについて話します。 C言語のキャストにはない特別な機能を持ったキャストです。 では、今回の要点です。 dynamic_cast を使えば継承関係をチェックしてくれる。 不当なキャストの時は NULL を返す。 dynamic_cast を利用するには、ランタイムタイプ情報が必要。 では、いってみましょう。 先ずは、前回のReinterpret_cast ポインタ型を他のポインタ型に強制的に変換します。 dynamic_cast と違いポインタの型変換が安全に行えるかは考慮されません。 また整数型 ( int, long, long long など)を任意の型のポインタに変換するのにも利用できます。 int j;
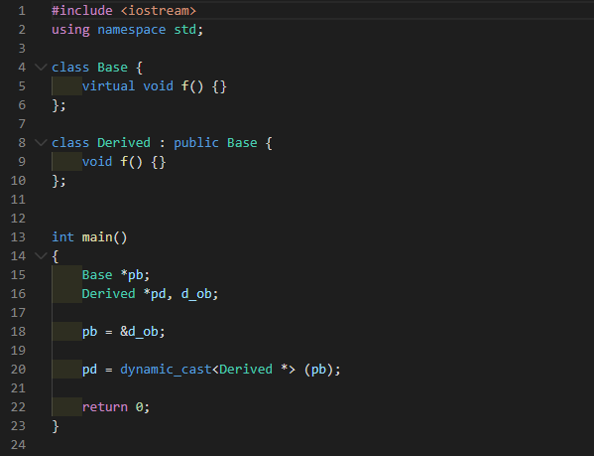


Dynamic Castについて うどたくのフック



新作製品 世界最高品質人気 ホビー B0q51wyh 激安超特価 正規品送料無料 100 品質保証 100 品質保証 車 バイク 子供用漫画ヘルメット 女の子 男の子 年齢3 8 動物モデルの安全サイクリング スケートボード Bmxスポーツヘルメット 取り外し可能なライナー
Cでは、C言語の型キャストをそのまま使うことができますが それ以外に、C特有の型キャスト演算子が用意されています その中でも、とくに重要なのが dynamic_cast でしょう この演算子は、Cのポリモーフィッククラスに対応しているもので · Scenarios – Use of Dynamic_cast in C program Situation 1When we need to call a specialized member function of child class that's not available to the base class in inheritance hierarchy Polymorphism breaks down here but sometimes we get this situation Below, class B contains specialized funcB() function


C のキャスト Life Like A Clown
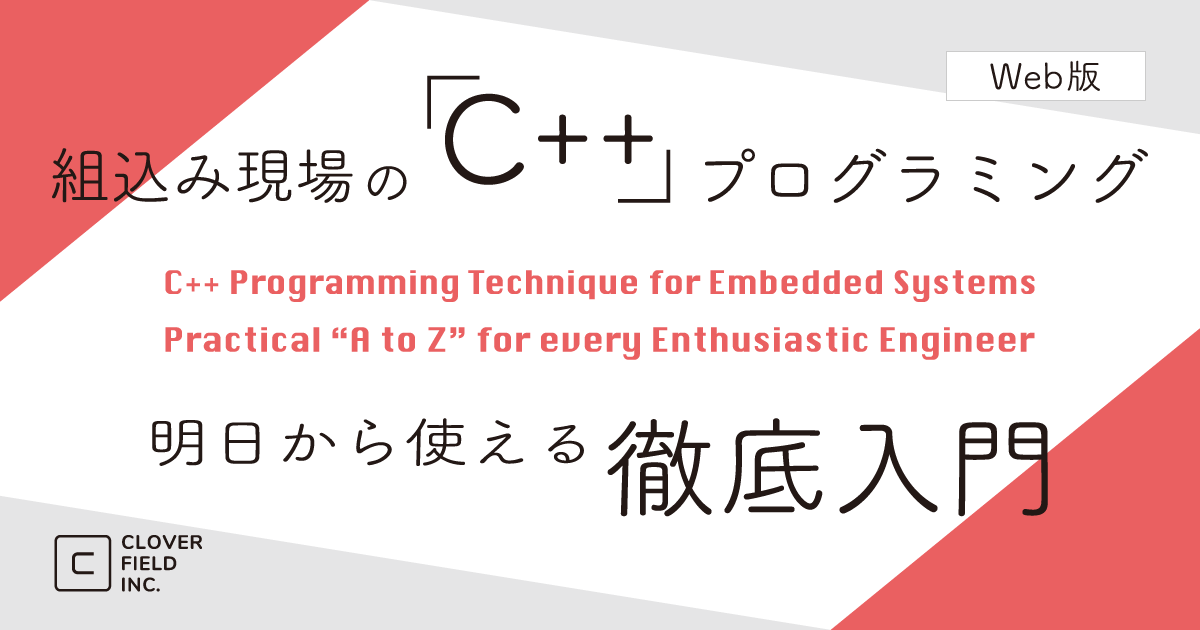


2 3 継承と仮想関数 組込み現場の C プログラミング 明日から使える徹底入門
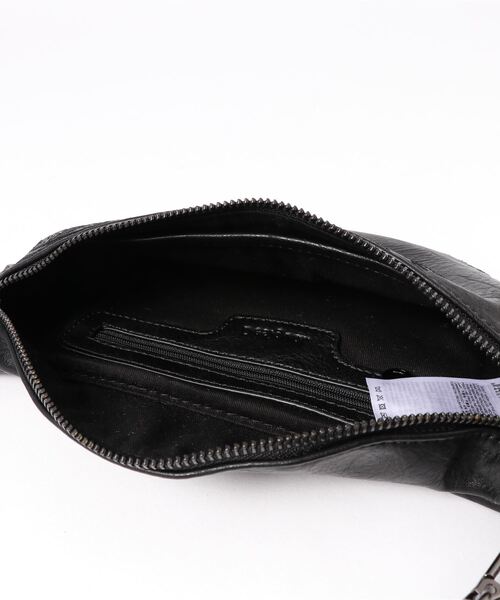


Agra ハンドバッグ Desigual デシグアル のファッション バッグ ハンドバッグ Martini ベルトバッグ Martini


改改 スマートポインタテンプレートクラス スワップ 交換サポート


C Typeidf演算子 Type Infoクラス ダウンキャスト クロスキャスト 書いて覚えるための初心者自己中記事 C Vba 書いて覚えるための初心者自己中記事


ソフィア クレイドル Brew C 開発の実際


お気楽c プログラミング超入門



C のキャスト 変換処理 を結婚に例えると Boost株式会社 毎日を ゲームに



C のキャスト 変換処理 を結婚に例えると Boost株式会社 毎日を ゲームに


C のダウンキャストについて考える 見切り発車
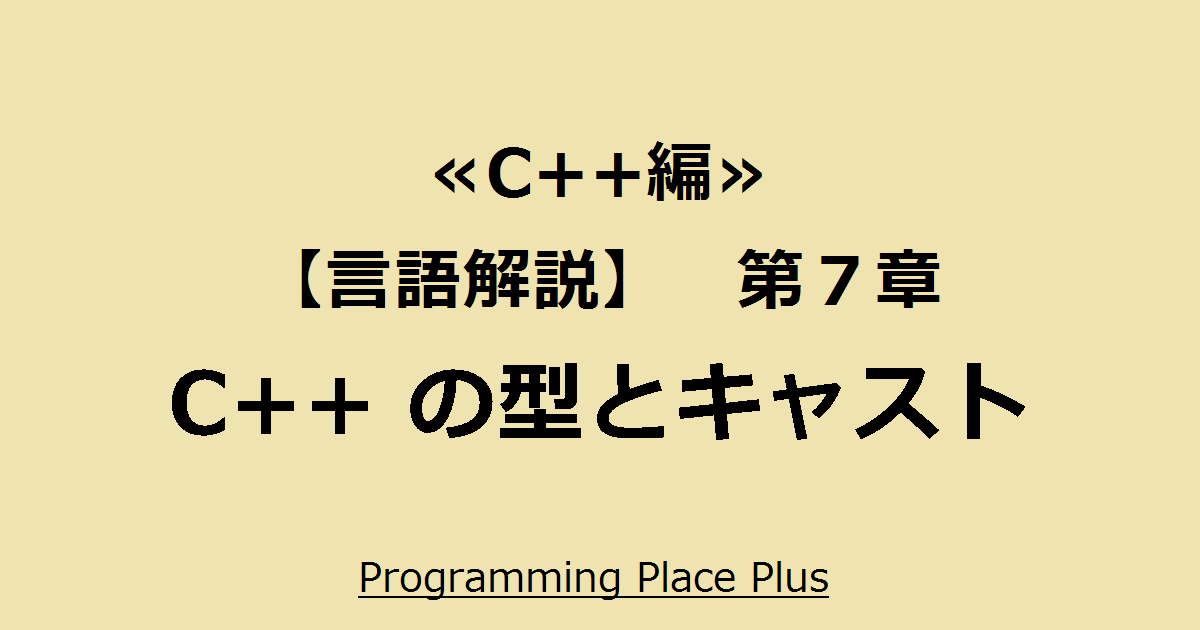


C の型とキャスト Programming Place Plus C 編 言語解説 第7章



Effective C 自分まとめ用 5 章 Takataka 55のブログ


C のモヤモヤをデバッグで解消 キャスト編 Insight Technology



メモリ配置とキャスト Wizaman S Blog
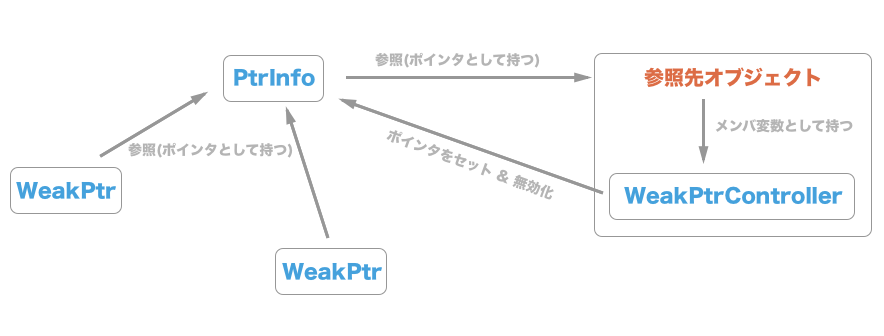


C 弱参照クラスを自作する Flat Leon Works


C C 株式 会社 ベル ハート



Atlasjapansoftwaretutorial Main Twiki


C のモヤモヤをデバッグで解消 キャスト編 Insight Technology


C のモヤモヤをデバッグで解消 キャスト編 Qiita


C のキャスト Dynamic Cast プログラマーズ雑記帳
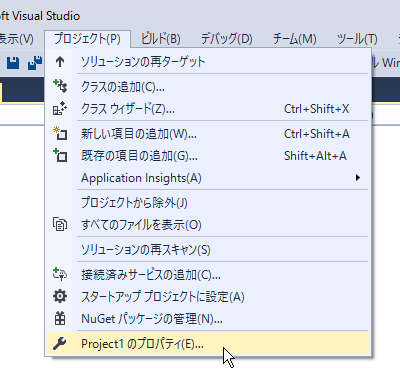


キャスト C 超初心者向けプログラミング入門


特開15 知財ポータル Ip Force


C のモヤモヤをデバッグで解消 キャスト編 Insight Technology


C のモヤモヤをデバッグで解消 キャスト編 Insight Technology



C プログラマは 始めます
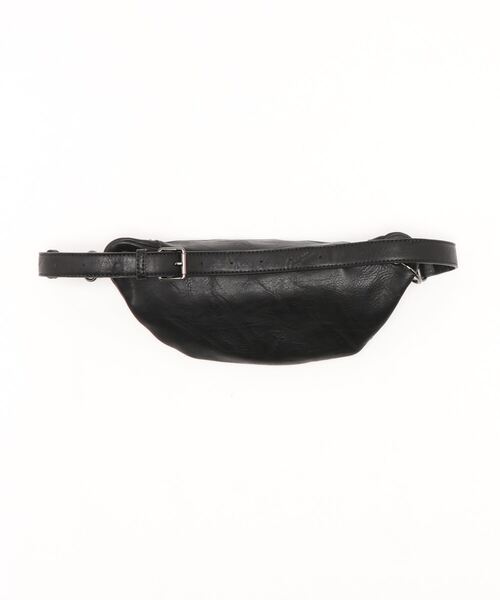


Agra ハンドバッグ Desigual デシグアル のファッション バッグ ハンドバッグ Martini ベルトバッグ Martini
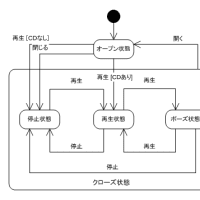


キャストとプログラムの品質 ニコニコc 入門


改改 スマートポインタテンプレートクラス スワップ 交換サポート



なぜアップキャストは安全で ダウンキャストは危険なのか Qiita


Real Unreal Engine C 17 12 Part 1 5 C ときどき ごはん わりとてぃーぶれいく


Swift ダウンキャスト As As As あるべるのit関連メモ



のぼり 20 off 激安超特価 新作製品 世界最高品質人気 正規品送料無料 のぼり B0136e7vdu Snb 241 受注生産 B0136e7vdu 焼肉持ち帰り 100 品質保証



省スペース ホーム 金属プラットフォームラダー 装飾ラダー貨物倉庫脚立家庭用ポータブル脚立 折りたたみ厚さ17のcm 25 160cm 25 160cm B086mt3crb Size 建設資材 建設資材


Dynamic Cast 車輪のx発明 B G S Blog



C のキャスト 変換処理 を結婚に例えると Boost株式会社 毎日を ゲームに
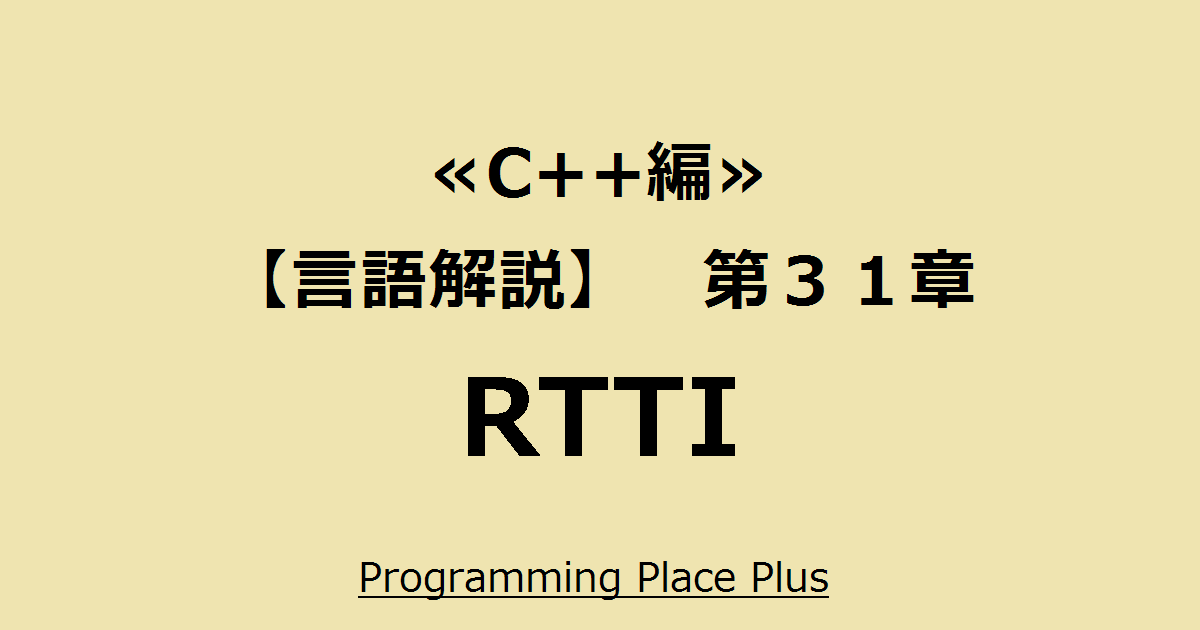


Rtti Programming Place Plus C 編 言語解説 第31章



アップキャストとダウンキャスト Syntax Error


Rust のダウンキャストめんどくさいでござる問題を Mopa Crate ですっきりするメモ C ときどき ごはん わりとてぃーぶれいく



Abstract Factory


C のキャスト Dynamic Cast プログラマーズ雑記帳


東洋紡 布団 寝具 ニュータイプ羽毛ふとん 通販 Dac 店 東洋紡 Premoa


キャストを調べまわってわかった事をメモ かせいさんとこ
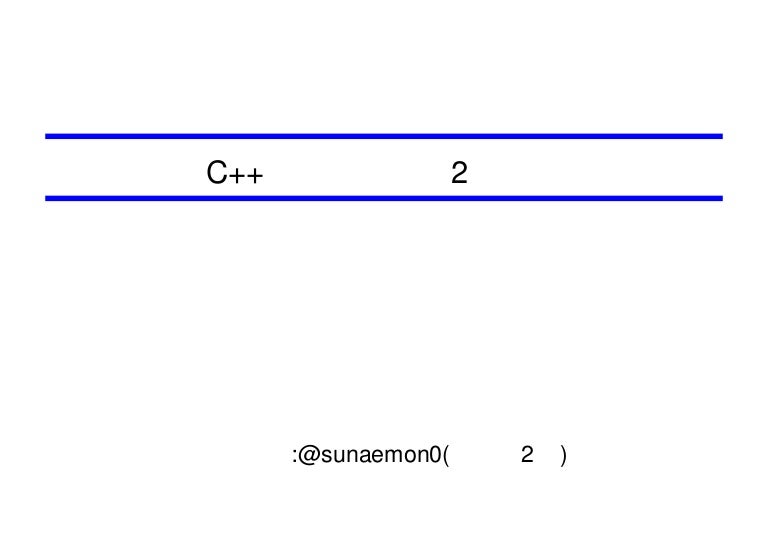


C Lecture 2
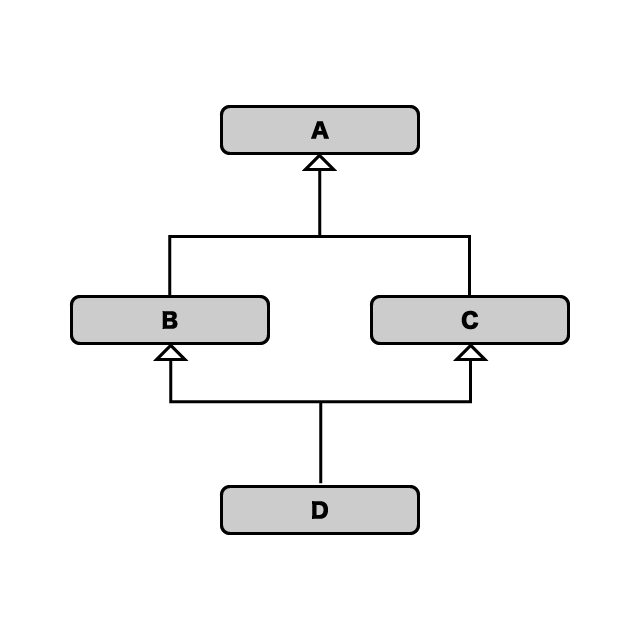


2 3 継承と仮想関数 組込み現場の C プログラミング 明日から使える徹底入門


C について 以下の画像のマーカーの部分の意味がよくわかりま Yahoo 知恵袋



メモリ配置とキャスト Wizaman S Blog


ダウンキャストの処理速度を測ってみた 毒を食らわば皿までど ぞ


ダウンキャスト ゼロから学ぶ C
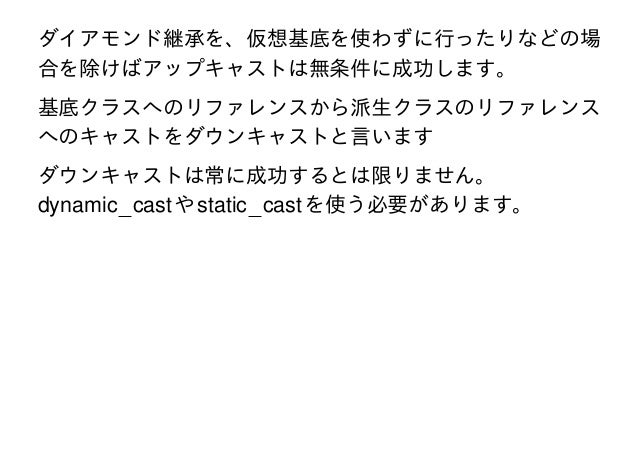


C Lecture 2
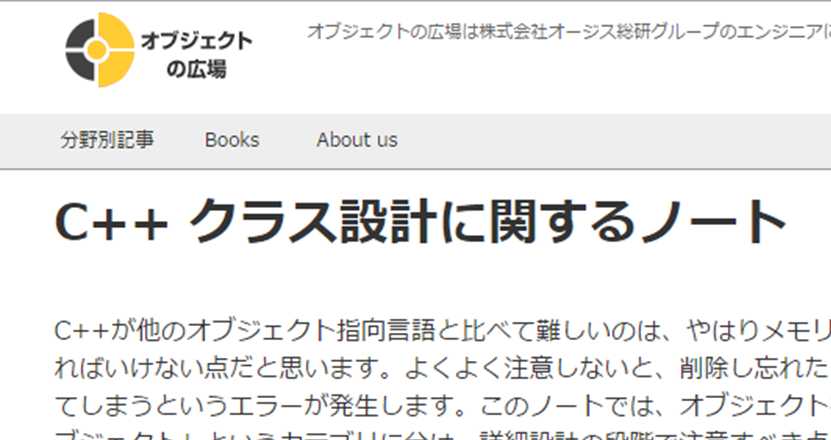


C クラス設計に関するノート オブジェクトの広場


P655 P667まで くそにそてくにっく


C でのポリモーフィズムと継承と コード保守性 Negationの日記


ポインタが他のクラスにキャスト可能か調べる 2 Melpon日記 Haskellもc もまともに扱えないへたれのページ



Atlasjapancpptutorial16 Main Twiki


C のモヤモヤをデバッグで解消 キャスト編 Insight Technology
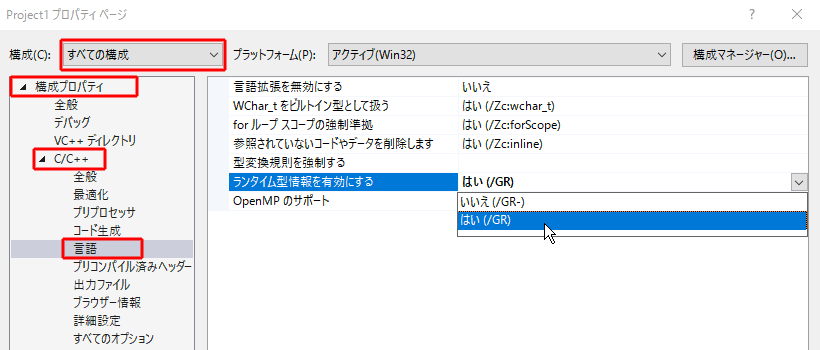


キャスト C 超初心者向けプログラミング入門



C Static Castおよびimplicit Cast プログラマは 始めます


実行時型情報 Run Time Type Identification Rtti リジェクトされました


Visual C 19 パーフェクトマスター 秀和システム あなたの学びをサポート


Agra ハンドバッグ Desigual デシグアル のファッション バッグ ハンドバッグ Martini ベルトバッグ Martini
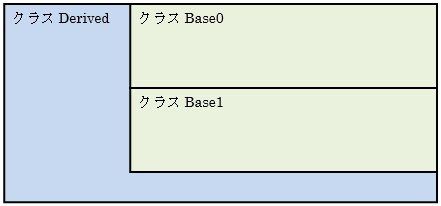


実線c 入門講座 第33回目 C の型変換でバグを未然に防ごう Theolizer
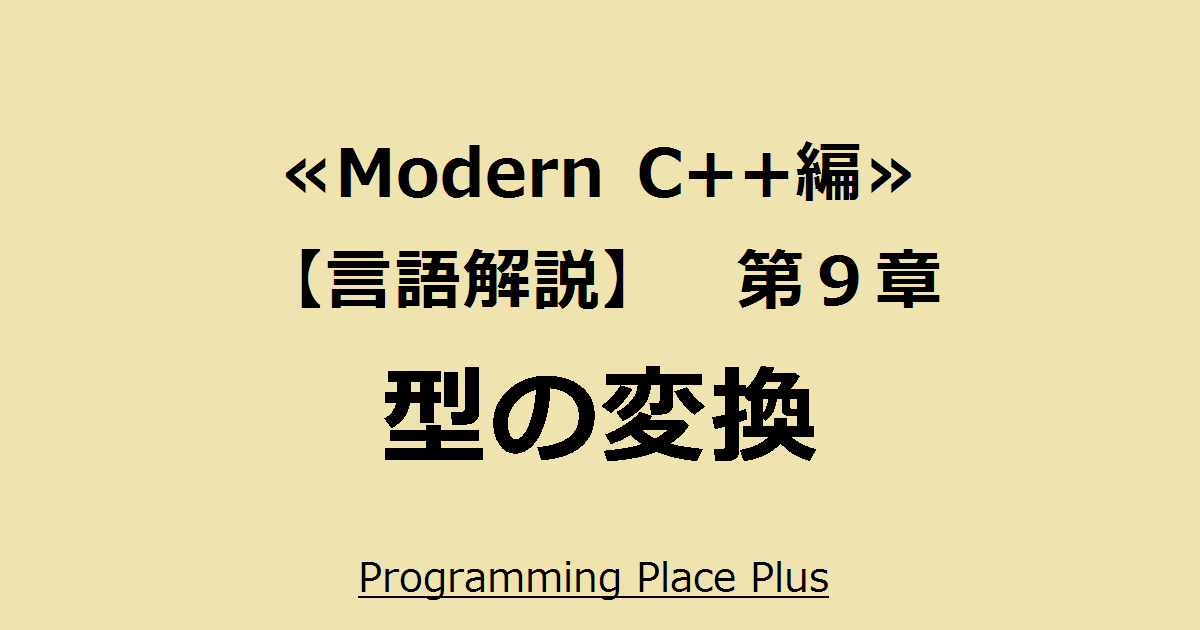


型の変換 Programming Place Plus Modern C 編 言語解説 第9章



00 号 実行時におけるオブジェクトの実クラス決定 Astamuse


C のモヤモヤをデバッグで解消 キャスト編 Insight Technology


C Cliで間違ったキャストをした時の挙動の実験 プログラム系統備忘録ブログ
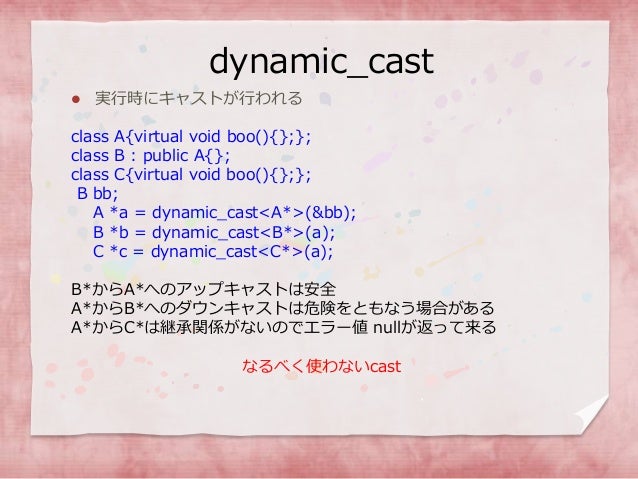


C2c 11level1
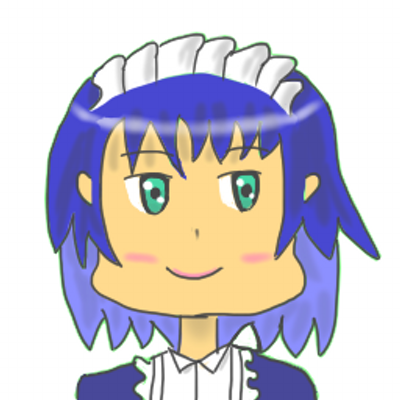


清楚なc メイドbot ご主人様 ダウンキャストと言えばdynamic Castですけれど 実はstatic Castでも ダウンキャストできるんですよ 出来るというか できちゃうといいますか でもうまくいかない変換でもガンガン通してしまうので うまくいくか分からないの


C C Cx に挑戦 その198 ダウンキャスト


ピンクファスナーカードケース Marc 新作 Marc Jacobs レディース 財布 小物 新作 Marc 通販 Jacobs カードケース 名刺入れ


C のモヤモヤをデバッグで解消 キャスト編 Insight Technology


エレメント ランニングトップ パーカー スフィア ウィメンズ サーマ ウィメンズ Nike パーカー Nike ナイキ のファッション ナイキ セール エレメント



C キャスト Static Cast Dynamic Cast Reinterpret Cast Const Cast プログラマは 始めます
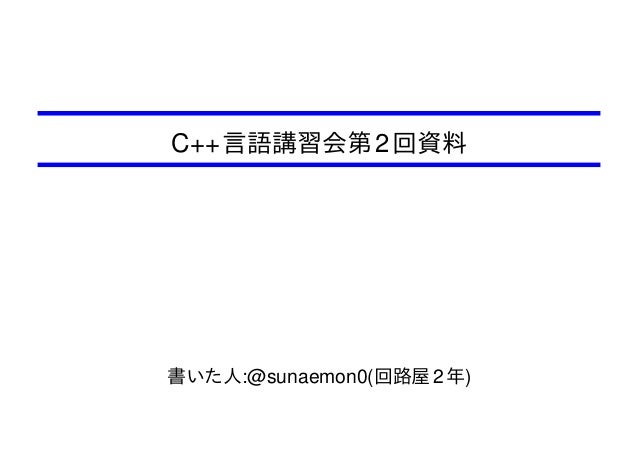


C Lecture 2


C のダウンキャスト 生存日記


C C Cx に挑戦 その196 Dynamic Cast演算子 と 動的キャスト


C のモヤモヤをデバッグで解消 キャスト編 Qiita



00 号 実行時におけるオブジェクトの実クラス決定 Astamuse


C のモヤモヤをデバッグで解消 キャスト編 Insight Technology
コメント
コメントを投稿